Authentication in Office Add-ins: Azure AD & MS Graph Explained
Implementing robust authentication mechanisms in Office Add-ins is crucial for ensuring secure and seamless user experiences. By leveraging Azure Active Directory (Azure AD) and the Microsoft Graph API, developers can provide users with single sign-on (SSO) capabilities and access to a wealth of Microsoft 365 data. This comprehensive guide explores the intricacies of authentication in Office Add-ins, detailing the integration of Azure AD and Microsoft Graph to build secure, efficient, and user-friendly applications.
Introduction to Office Add-ins Authentication
Office Add-ins extend the functionality of Microsoft Office applications by integrating web-based features directly into the user interface. As part of delivering Custom Microsoft Office Solutions, these add-ins often need to interact with user data securely and provide personalized experiences. To achieve this, they must implement robust authentication mechanisms. Azure AD serves as the primary identity provider, enabling developers to authenticate users and access Microsoft 365 services through the Microsoft Graph API.
Understanding Azure Active Directory (Azure AD)
Azure Active Directory is Microsoft’s cloud-based identity and access management service. It allows developers to authenticate users, enforce security policies, and access organizational resources. Integrating Azure AD into Office Add-ins ensures that authentication processes align with enterprise security standards and provide seamless access to Microsoft services.
Overview of Microsoft Graph API
The Microsoft Graph API is a unified endpoint that enables developers to access data and intelligence from Microsoft 365 services. By integrating Microsoft Graph into Office Add-ins, developers can retrieve user profiles, access mail and calendar data, manage files in OneDrive, and more, providing rich functionalities within their applications.
Authentication Flows in Office Add-ins
Implementing authentication in Office Add-ins can be achieved through various flows, each catering to different scenarios and requirements.
Single Sign-On (SSO) with Azure AD
Single Sign-On (SSO) allows users to authenticate once and gain access to multiple applications without repeated sign-ins. In the context of Office Add-ins, SSO leverages the user’s existing Office session to authenticate seamlessly.
How SSO Works in Office Add-ins:
- User Authentication: The add-in requests an access token by calling the OfficeRuntime.auth.getAccessToken method.
- Token Acquisition: Office communicates with Azure AD to obtain an access token for the add-in.
- Access Token Usage: The add-in uses the access token to authenticate API calls to its own server or to Microsoft Graph.
This process eliminates the need for users to re-enter credentials, enhancing user experience and security.
Fallback Authentication Using the Office Dialog API
In scenarios where SSO is unavailable or fails (e.g., due to configuration issues or user policies), the Office Dialog API provides a fallback authentication method.
Fallback Authentication Process:
- Dialog Invocation: The add-in opens a dialog window using Office.context.ui.displayDialogAsync.
- User Sign-In: The dialog loads the authentication page, prompting the user to sign in.
- Token Retrieval: Upon successful authentication, the dialog retrieves the access token and passes it back to the add-in.
This approach ensures that users can still authenticate and use the add-in’s features when SSO is not an option.
Registering Your Add-in with Azure AD
To integrate Azure AD authentication into your Office Add-in, you must register the add-in as an application in Azure AD.
Steps to Register:
- Access Azure Portal: Navigate to the Azure portal and sign in with administrative credentials.
- App Registration:
- Go to Azure Active Directory > App registrations > New registration.
- Provide a name for your application.
- Set the Supported account types based on your target audience.
- Specify the Redirect URI, typically in the format https://your-domain/auth/callback.
3. Configure API Permissions:
- In the app’s API permissions section, add permissions required for Microsoft Graph (e.g., User.Read, Mail.Read).
- Grant admin consent if necessary.
4. Expose an API:
- Define the scopes that your add-in will use.
- Set the Application ID URI.
This registration process establishes a trust relationship between your add-in and Azure AD, enabling secure authentication and authorization.
Implementing SSO in Your Office Add-in
With the add-in registered, you can implement SSO to authenticate users seamlessly.
Client-Side Implementation:
1. Token Acquisition:
- Call OfficeRuntime.auth.getAccessToken to obtain an access token.
- Handle scenarios where the token is unavailable or expired.
2. Token Validation:
Once you receive the access token on the client side:
javascript code:
OfficeRuntime.auth.getAccessToken({ allowSignInPrompt: true });
Secure Your Office Add-In with Azure AD & Microsoft Graph
Looking to implement robust authentication in your Office Add-In? Schedule a consultation with MDS to integrate Azure AD and Microsoft Graph seamlessly.
Send it to your backend (Node.js, .NET, etc.) for validation. On the server:
- Use a library like jsonwebtoken (Node.js) or Microsoft.IdentityModel.Tokens (.NET).
Validate:
- Signature
- Issuer (https://sts.windows.net/{tenantid}/)
- Audience (your client_id)
- Token expiration
3. Secure API Calls:
After validating the token, your backend can now make secure requests to Microsoft Graph on behalf of the user, using either:
- Delegated Permissions: Use access token from getAccessToken()
- Application Permissions: Authenticate with client credentials (for background tasks)
Accessing Microsoft Graph API from Your Add-in
With authentication in place, use the Microsoft Graph API to access a user’s data and services.
1. Set Up Microsoft Graph SDK
Install Microsoft Graph client SDK in your backend or frontend (if using SPA flow):
bash code:
npm install @microsoft/microsoft-graph-client
npm install @microsoft/microsoft-graph-client
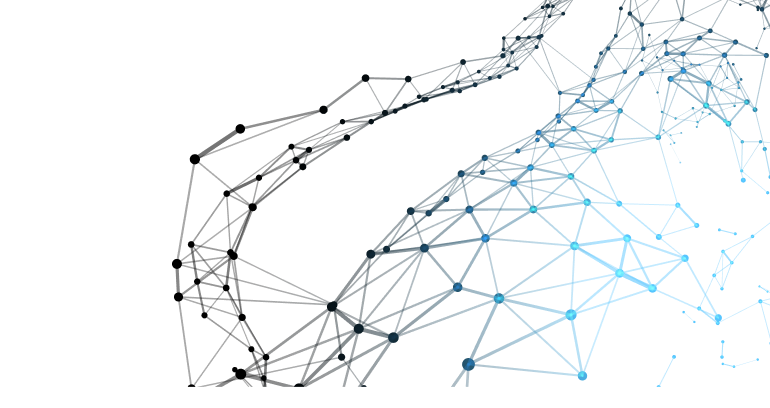
Struggling with Authentication in Office Add-ins? Let’s Simplify It!
Seamlessly integrate Azure Active Directory and Microsoft Graph to power secure, scalable, and user-friendly Office Add-ins. From token acquisition to Graph API access—we help you get it right.
🧠 As a trusted Microsoft 365 development partner, we ensure smooth authentication flows, compliance with best practices, and performance optimization.
📩 Contact us today for a free consultation and take the complexity out of Office Add-in authentication!
2. Initialize the Graph Client
javascript code:
const client = MicrosoftGraph.Client.init({
authProvider: (done) => {
done(null, accessToken);
},
});
3. Example: Fetch User Profile
javascript code:
const user = await client.api('/me').get();
console.log(user.displayName);
4. Other Popular Graph Endpoints for Add-ins
Use Case – Endpoint
- Get user’s profile – /me
- Get mail folders – /me/mailFolders
- Read inbox messages – /me/messages
- Access calendar events – /me/events
- Upload file to OneDrive – /me/drive/root:/filename:/content
- Search Teams messages – /me/chats or /teams/{id}
Make sure your app has been granted the appropriate Graph API scopes in Azure AD, and that consent is granted by the user or admin.
Handling Fallback Authentication with Dialog API
If SSO fails (e.g., user not signed into Office, token issues), use the Office Dialog API to implement interactive login.
1. Trigger the Dialog
javascript code:
Office.context.ui.displayDialogAsync(
"https://yourdomain.com/auth/login.html",
{ height: 60, width: 40 },
(result) => {
const dialog = result.value;
dialog.addEventHandler(Office.EventType.DialogMessageReceived, (message) => {
const token = message.message;
dialog.close();
});
}
);
2. Use MSAL.js in Login Page
In the dialog page (login.html), implement login using MSAL.js v2:
javascript code:
const msalInstance = new msal.PublicClientApplication({
auth: {
clientId: '',
authority: 'https://login.microsoftonline.com/common/',
redirectUri: 'https://yourdomain.com/auth/login.html',
},
});
msalInstance.loginPopup({ scopes: ['User.Read'] }).then(response => {
Office.context.ui.messageParent(response.accessToken);
});
This is especially important for Outlook Desktop, where SSO is not always guaranteed.
Best Practices for Secure Authentication in Office Add-ins
Security Practices
- Always validate tokens server-side before trusting user identity
- Use HTTPS for all API endpoints and resources
- Avoid storing tokens in localStorage; use secure cookies or session memory
Manifest & Permission Configuration
- Add the SingleSignOn requirement set in manifest.xml:
xml code:
Define WebApplicationInfo for SSO:
xml code:
your-client-id
api://your-client-id
User Experience Tips
- Provide a fallback authentication flow to avoid total failure
- Show clear error messages when token acquisition fails
- Offer “Sign out” options when using persistent login
- Use MSAL.js v2 (SPA support) for better SSO handling
Token Lifecycle Management
- Access tokens expire (typically in 1 hour); implement token refresh logic
- Use silent token acquisition when possible
- Catch and handle interaction_required errors in MSAL
Real-World Scenarios for Azure AD + Graph Integration
- Personalized dashboards in Excel using /me data
- Outlook Add-in that reads the selected message and summarizes using OpenAI
- Meeting room suggestions using /me/calendar/events
- Word Add-in that fetches company HR data via Graph + SharePoint
- Automate file upload workflows using OneDrive Graph endpoints
Conclusion
Integrating authentication in Office Add-ins using Azure Active Directory and Microsoft Graph API is essential for building secure, intelligent, and user-friendly solutions.
By leveraging SSO, MSAL, and Microsoft Graph, developers can:
- Authenticate users securely without disrupting workflows
- Access Microsoft 365 services such as Mail, Calendar, Teams, and OneDrive
- Deliver seamless, personalized, and enterprise-ready Office experiences
- Align with organizational identity and access management policies
Whether you’re building an enterprise Outlook plugin, an Excel dashboard tool, or a Word document assistant, understanding authentication is foundational to success in the Microsoft 365 ecosystem.
Related Hashtags:
#OfficeAddins #AzureAD #MSGraph #Microsoft365Dev #MSAL #OfficeJS #SSO #Authentication #OfficeDev #ReactAddins #OfficeSecurity #GraphAPI